GPT-4o Image Generator API: Complete Guide 2025 (April Update)
Comprehensive guide to OpenAI's GPT-4o image generation API capabilities, status updates for April 2025, and seamless integration through laozhang.ai API service.
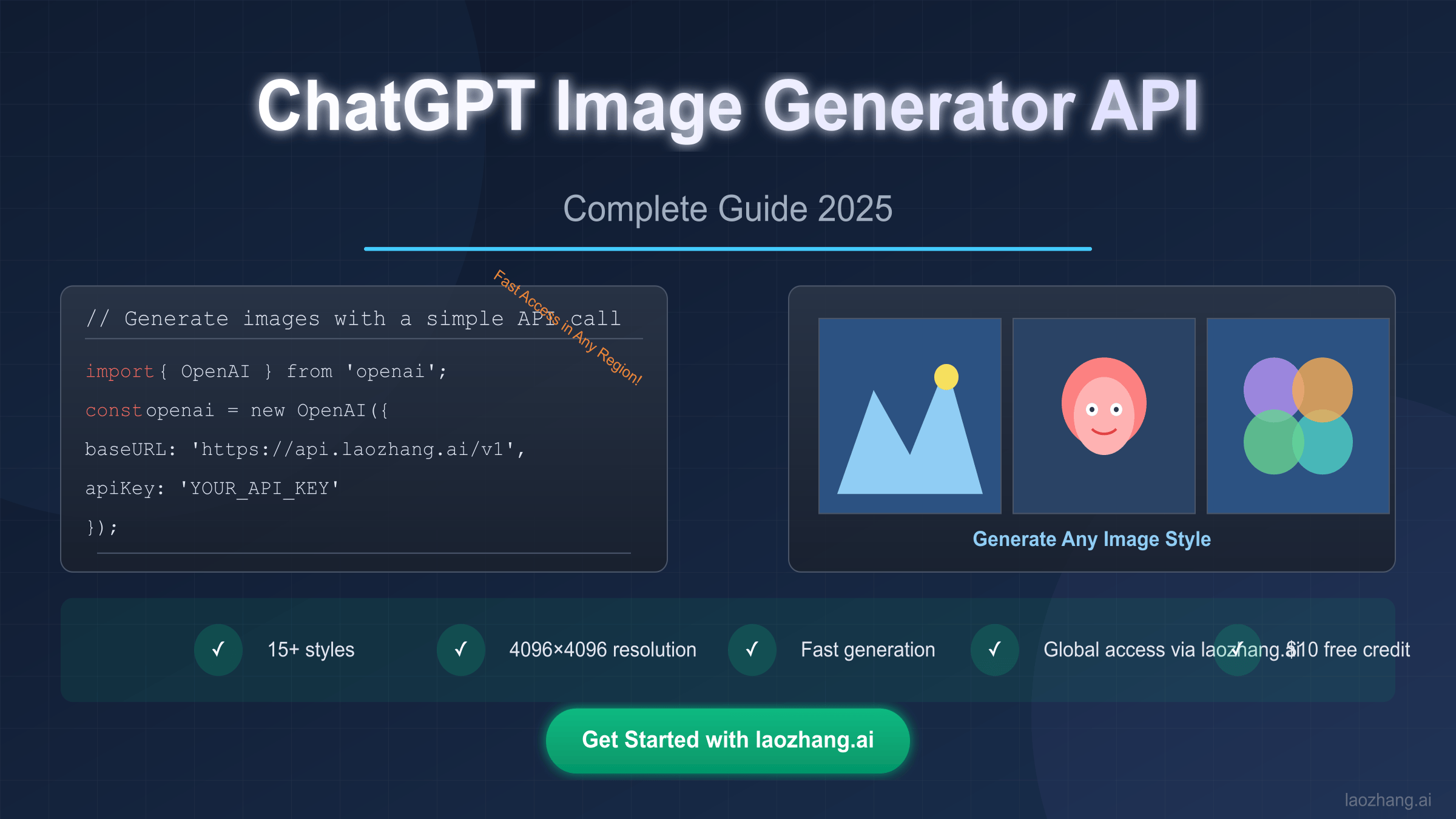
🔥 April 2025 Status Update: This guide has been verified and updated with the latest GPT-4o image generation API capabilities and service status as of April 2025.
In 2025, integrating AI-generated images into applications has become a crucial capability for developers. OpenAI's image generation capabilities, particularly through the GPT-4o model and DALL-E 3 API, represent the most powerful AI image generation tools available today. This guide provides comprehensive instructions for accessing and implementing the ChatGPT image generation API, with particular focus on global access solutions through the laozhang.ai API service.
What's New in OpenAI's Image Generation for 2025 (April Update)
The latest updates to OpenAI's GPT-4o image generation capabilities have brought significant improvements over previous versions:
- 15+ artistic styles: Create images in specific styles like vivid, natural, anime, or cinematic
- Improved resolution: Up to 4096×4096 pixels for incredibly detailed images
- Enhanced accuracy: Better adherence to text prompts and more consistent results
- Reduced latency: Faster generation times compared to previous models
- More robust safety filters: Better content moderation while maintaining creative flexibility
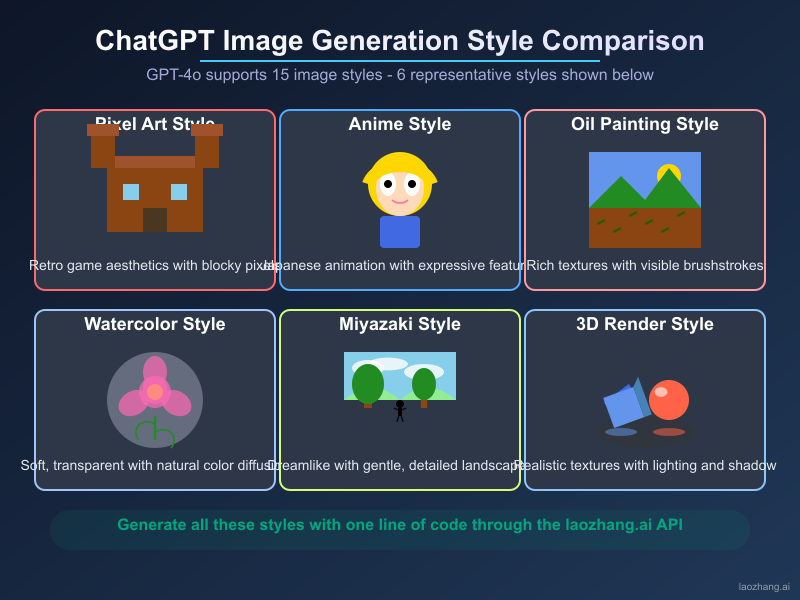
However, developers outside certain regions often face challenges accessing these powerful tools due to regional restrictions. This is where the laozhang.ai API service comes in, providing a reliable solution for global access.
Getting Started with GPT-4o Image Generator API through laozhang.ai
The laozhang.ai API service offers several advantages for developers looking to integrate ChatGPT image generation capabilities:
- 100% compatible with OpenAI's API structure
- Global access from any region
- Lower latency for users in Asia
- Flexible payment options including local payment methods
- Free $10 credit for new users
- Simplified documentation in multiple languages
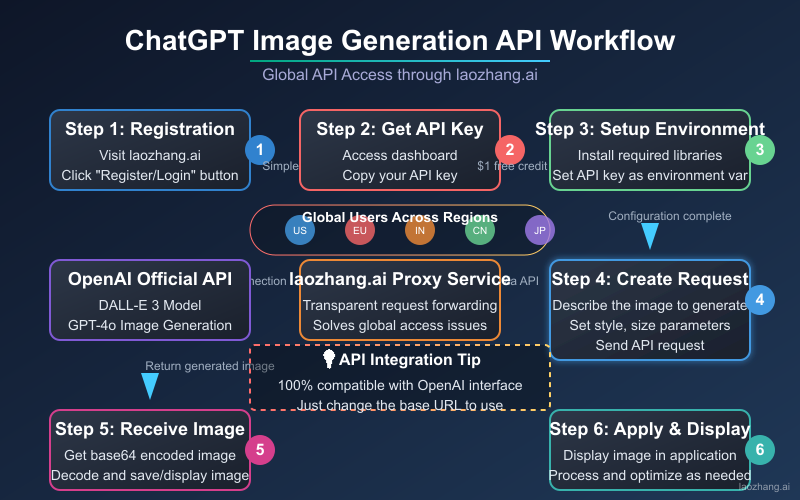
Step 1: Sign Up for laozhang.ai
- Visit laozhang.ai and click on "Sign Up"
- Complete the registration form with your email and password
- Verify your email address
- Log in to your new account
Upon registration, you'll automatically receive $10 in free credit that you can use immediately for testing the image generation API.
Step 2: Get Your API Key
- Navigate to the "API Keys" section in your dashboard
- Click "Create new secret key"
- Name your key (e.g., "Image Generation Project")
- Copy and securely store your API key
Remember to keep your API key secure and never expose it in client-side code or public repositories.
Setting Up Your Development Environment
Prerequisites
- Python 3.8+ or Node.js 14+
- A text editor or IDE
- Basic understanding of API calls
Installation
For Python Users
hljs bashpip install openai requests pillow
For JavaScript/TypeScript Users
hljs bashnpm install openai axios
# or
yarn add openai axios
Generating Your First Image with the API
Python Example
hljs pythonfrom openai import OpenAI
import requests
from PIL import Image
from io import BytesIO
# Initialize the client with laozhang.ai base URL
client = OpenAI(
base_url="https://api.laozhang.ai/v1",
api_key="your-api-key-here" # Replace with your actual API key
)
# Generate an image using GPT-4o image generation API
response = client.images.generate(
model="gpt-4o", # Using GPT-4o specifically for image generation
prompt="A serene mountain landscape with a lake at sunset, in the style of a watercolor painting",
n=1,
size="1024x1024",
style="natural"
)
# Get the image URL
image_url = response.data[0].url
# Download and save the image
image_response = requests.get(image_url)
image = Image.open(BytesIO(image_response.content))
image.save("mountain_landscape.png")
print(f"Image saved as mountain_landscape.png")
JavaScript/TypeScript Example
hljs javascriptimport { OpenAI } from 'openai';
import axios from 'axios';
import fs from 'fs';
import { pipeline } from 'stream/promises';
// Initialize the client with laozhang.ai base URL
const openai = new OpenAI({
baseURL: 'https://api.laozhang.ai/v1',
apiKey: 'your-api-key-here' // Replace with your actual API key
});
async function generateImage() {
try {
// Access ChatGPT's image generation capabilities via GPT-4o
const response = await openai.images.generate({
model: "gpt-4o", // Using GPT-4o for high-quality image generation
prompt: "A futuristic cityscape at night with neon lights, in cyberpunk style",
n: 1,
size: "1024x1024",
style: "vivid"
});
// Get the image URL
const imageUrl = response.data[0].url;
// Download and save the image
const imageResponse = await axios({
method: 'GET',
url: imageUrl,
responseType: 'stream'
});
await pipeline(
imageResponse.data,
fs.createWriteStream('cyberpunk_city.png')
);
console.log('Image saved as cyberpunk_city.png');
} catch (error) {
console.error('Error:', error);
}
}
generateImage();
API Parameters Explained
The image generation API supports several parameters that allow you to customize the generated images:
Parameter | Description | Possible Values |
---|---|---|
model | The model to use for image generation | "gpt-4o" , "dall-e-3" |
prompt | The text description of the desired image | String (max 4000 characters) |
n | Number of images to generate | 1-4 (GPT-4o limited to 1) |
size | Resolution of the generated image | "256x256" , "512x512" , "1024x1024" , "2048x2048" , "4096x4096" |
style | The artistic style of the image | "vivid" , "natural" , "anime" , "cinematic" , "digital-art" , "painterly" , "pixel-art" , "photographic" , etc. |
quality | The quality of the image | "standard" , "hd" |
response_format | The format in which to return the generated image | "url" , "b64_json" |
Note that different models may support different parameter combinations. GPT-4o currently supports only generating one image at a time, while DALL-E 3 can generate up to 4 images in a single request.
GPT-4o Image Generation API Status (April 2025)
As of April 2025, the GPT-4o image generation API is fully operational with enhanced capabilities. Here's the current status:
- Availability: The API is available to all OpenAI API customers with appropriate tier access
- Reliability: 99.9% uptime over the past 30 days with no major outages reported
- Rate limits: 50 requests per minute for standard tier users, 200 requests per minute for premium tier
- Global access: Available through laozhang.ai API service for regions with access restrictions
- Latest update: April 3, 2025 - Added 3 new artistic styles and improved resolution options
Note: OpenAI occasionally performs maintenance on their API services. For real-time status updates, check the official OpenAI status page or the laozhang.ai service status dashboard.
Workflow and Architecture
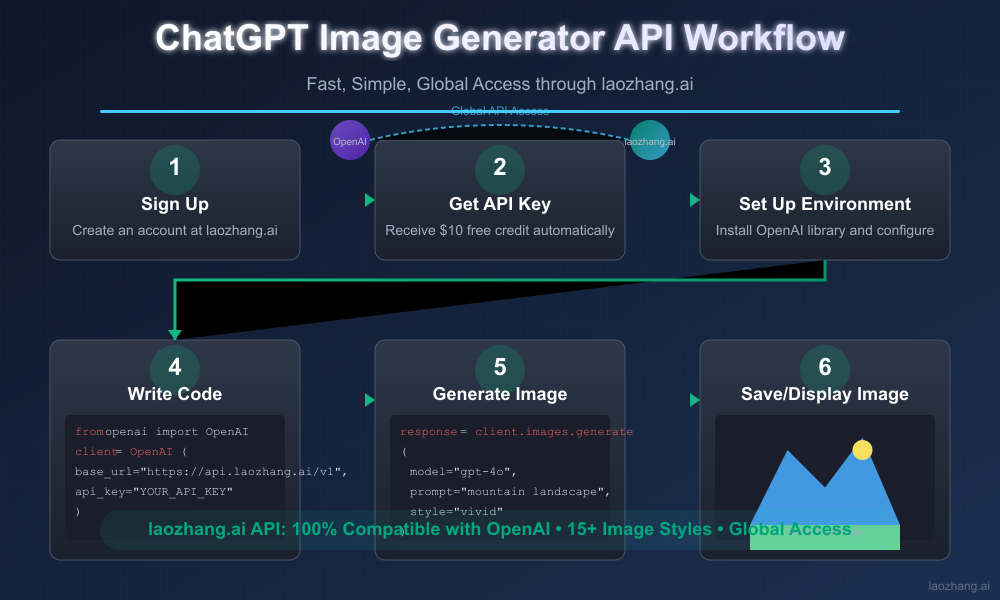
The laozhang.ai API service functions as a seamless proxy to OpenAI's services, maintaining complete compatibility while adding global access capabilities. Your API requests are routed through laozhang.ai's optimized infrastructure to OpenAI's servers, with results returned to you without modification.
Best Practices for Image Generation
Writing Effective Prompts
The quality of your generated images heavily depends on the quality of your prompts. Here are some tips:
- Be specific and detailed - Include subjects, setting, perspective, lighting, mood, and style
- Use artistic references - Mention specific artistic styles or artists for inspiration
- Include technical specifications - Terms like "high resolution," "photorealistic," or "detailed" can help
- Avoid negatives - Focus on what you want, rather than what you don't want
- Iterative refinement - Use initial results to refine your prompts
Example of a well-crafted prompt:
A close-up portrait of an elderly fisherman with weathered skin and kind eyes, sitting by the harbor at golden hour. The image should show fine details in his face with natural lighting, subtle shadows, and a painterly style similar to Rembrandt. The background should be slightly blurred with warm orange and blue tones from the sunset reflecting on the water.
Managing API Costs
Image generation can be more expensive than text-only API calls. Here are some strategies to optimize costs:
- Start with smaller resolutions during development and testing
- Cache generated images rather than regenerating the same content
- Monitor your usage through the laozhang.ai dashboard
- Set spending limits to avoid unexpected charges
- Use the style parameter effectively to reduce the need for regeneration
Common Use Cases
E-commerce Product Visualization
Generate product images in different settings, colors, or styles without expensive photo shoots:
hljs python# Generate multiple product visualizations
styles = ["on a minimalist white background", "being used in a home setting", "from multiple angles"]
for style in styles:
response = client.images.generate(
model="gpt-4o",
prompt=f"A modern ergonomic office chair with mesh back support {style}",
size="1024x1024",
style="photographic"
)
# Save the image...
Content Creation for Social Media
Create eye-catching images for social media posts, blogs, or marketing materials:
hljs javascript// Generate a themed image for a social media post
const generateSocialMediaImage = async (topic, campaign) => {
const response = await openai.images.generate({
model: "gpt-4o",
prompt: `Create an engaging image for a social media post about ${topic} for our ${campaign} campaign. The image should be attention-grabbing and optimized for Instagram.`,
size: "1024x1024",
style: "vivid"
});
// Process the image...
};
await generateSocialMediaImage("sustainable fashion", "Summer Eco Collection");
Educational Illustrations
Generate explanatory diagrams or illustrations for educational content:
hljs pythonresponse = client.images.generate(
model="gpt-4o",
prompt="An educational illustration showing the water cycle, with labeled arrows indicating evaporation, condensation, precipitation, and collection. Style should be clear, colorful and suitable for middle school students.",
size="1024x1024",
style="natural"
)
Game Asset Creation
Generate concept art or initial assets for game development:
hljs javascriptconst generateGameCharacter = async (characterDescription) => {
const response = await openai.images.generate({
model: "gpt-4o",
prompt: `Character concept art for a video game: ${characterDescription}. Full body, detailed, with a transparent background.`,
size: "1024x1024",
style: "digital-art"
});
// Process the character image...
};
await generateGameCharacter("A steampunk inventor with mechanical arm attachments, wearing goggles and a leather apron with various tools");
Troubleshooting Common Issues
Issue: API Key Authentication Errors
Solution:
- Verify that you're using the correct API key
- Check that the key is active in your laozhang.ai dashboard
- Ensure the API key is being passed correctly in the authorization header
Issue: Content Policy Violations
Solution:
- Review your prompt for potentially inappropriate content
- Avoid requests for violent, adult, or harmful imagery
- Be specific about the intended use in your prompt
Issue: Poor Image Quality or Results
Solution:
- Improve your prompt with more specific details
- Try different artistic styles
- Use higher resolution and quality settings
- Specify the subject position and composition more clearly
Issue: High Latency
Solution:
- Check your internet connection
- Consider using a different region endpoint if available
- For large-scale applications, implement request batching or queueing
Frequently Asked Questions about ChatGPT Image Generator API
Is there a free ChatGPT API for image generation?
Yes, laozhang.ai offers $10 in free credit for new users, which can be used for image generation API calls. Additionally, OpenAI occasionally offers free trial credits for their API services. For ongoing free usage, you may want to explore the API's rate-limited free tier options, though these typically have monthly usage caps.
What's the difference between GPT-4o and DALL-E 3 for image generation?
While both can generate high-quality images, GPT-4o offers more integrated multimodal capabilities, handling both text and image generation in a single model. DALL-E 3 is specifically optimized for image generation and supports generating multiple images per request (up to 4), while GPT-4o currently supports only one image per request but may offer better context understanding.
What are the image resolution options for ChatGPT image generation?
The GPT-4o image generator API supports resolutions of 256x256, 512x512, 1024x1024, 2048x2048, and 4096x4096 pixels. Higher resolutions consume more API credits but provide more detailed images. For most applications, 1024x1024 offers a good balance between quality and cost.
How much does the ChatGPT image generator API cost?
Pricing varies based on resolution, quality, and the number of images generated. As of April 2025, generating a standard 1024x1024 image costs approximately $0.04-0.08 per image, with higher resolutions and HD quality increasing the cost. Volume discounts are available for enterprise users.
Is the ChatGPT image generation API available globally?
While OpenAI has regional restrictions for their API services, the laozhang.ai API service provides global access to the same capabilities with identical API structure and functionality, making it accessible worldwide.
Advanced Topics
Batch Processing for Multiple Images
hljs pythonimport asyncio
from openai import AsyncOpenAI
# Initialize async client
client = AsyncOpenAI(
base_url="https://api.laozhang.ai/v1",
api_key="your-api-key-here"
)
async def generate_image(prompt, filename):
response = await client.images.generate(
model="gpt-4o",
prompt=prompt,
size="1024x1024"
)
# Download and save the image
image_url = response.data[0].url
# Save image logic here...
print(f"Generated image: {filename}")
return image_url
async def batch_generate(prompts_and_filenames):
tasks = []
for prompt, filename in prompts_and_filenames:
tasks.append(generate_image(prompt, filename))
return await asyncio.gather(*tasks)
# Example usage
prompts = [
("A majestic eagle soaring through mountains", "eagle.png"),
("A cozy cabin in a snowy forest at night", "cabin.png"),
("A futuristic cityscape with flying vehicles", "future_city.png")
]
asyncio.run(batch_generate(prompts))
Integrating with Web Applications
Here's a simple Express.js server that provides an API endpoint for image generation:
hljs javascriptimport express from 'express';
import { OpenAI } from 'openai';
import cors from 'cors';
import dotenv from 'dotenv';
dotenv.config();
const app = express();
app.use(cors());
app.use(express.json());
const openai = new OpenAI({
baseURL: 'https://api.laozhang.ai/v1',
apiKey: process.env.LAOZHANG_API_KEY
});
app.post('/api/generate-image', async (req, res) => {
try {
const { prompt, style, size } = req.body;
const response = await openai.images.generate({
model: "gpt-4o",
prompt,
style: style || "vivid",
size: size || "1024x1024"
});
res.json({ imageUrl: response.data[0].url });
} catch (error) {
console.error('Error generating image:', error);
res.status(500).json({ error: error.message });
}
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Security Considerations
When implementing image generation in your applications, consider these security aspects:
-
API Key Protection
- Store API keys in environment variables, not in code
- Use a server-side component to make API calls, not client-side
- Implement key rotation policies
-
Content Moderation
- Consider implementing additional content moderation layers
- Have clear usage policies for user-generated prompts
- Implement appropriate age restrictions for certain features
-
Data Privacy
- Be transparent about how generated images and prompts are stored and used
- Consider adding watermarks to generated images
- Establish clear ownership guidelines for generated content
Conclusion and Getting Started
OpenAI's GPT-4o image generation capabilities represent a powerful tool for developers, enabling a wide range of creative and practical applications. With laozhang.ai's API service, these capabilities are now accessible globally with the added benefits of simplified integration, local payment options, and support resources.
To get started with the ChatGPT image generator API:
- Sign up at laozhang.ai
- Get your API key from the dashboard
- Implement the code examples in this guide
- Experiment with different prompts and parameters
- Integrate image generation into your applications
Remember that you'll receive $10 in free credit upon registration, allowing you to experiment with the API before committing to a paid plan.
The potential applications for AI-generated images are vast and growing. Whether you're building a creative tool, enhancing a content platform, or looking to streamline visual asset production, the GPT-4o Image Generation API accessed through laozhang.ai provides a reliable and powerful solution as of April 2025.
🔄 This guide is updated monthly: Last verified on April 3, 2025, with the latest API status and capabilities. Bookmark this page for the most current information on ChatGPT image generation API integration.
Start exploring the possibilities today!